API Integration
In your Xendit Dashboard, go to Settings > API Key and generate a Secret Key.
- Enter any API key name for your reference
- Set WRITE permission for Money-In
- Click Generate key
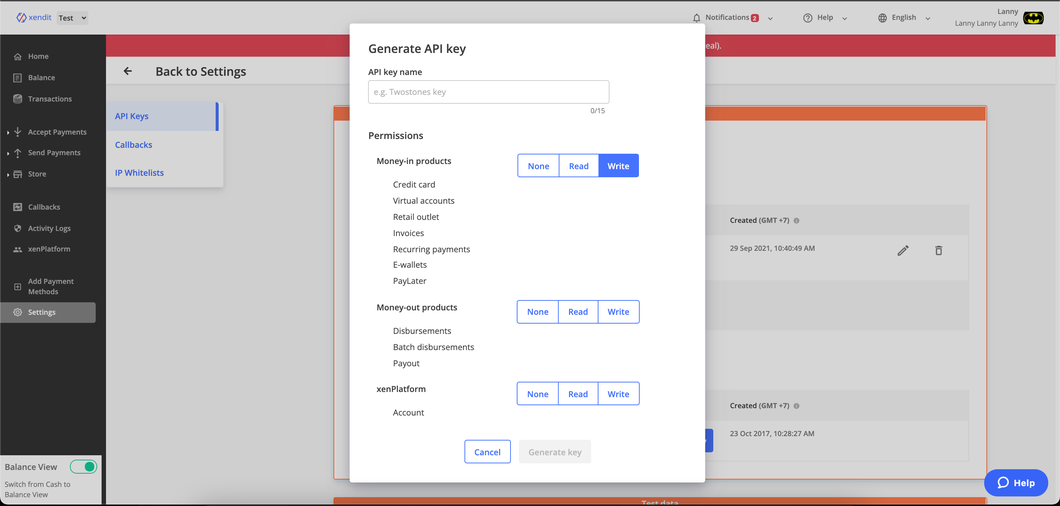
INFO
For testing environment, generate API Key on Test Mode. After testing, you need to create another API Key on Live Mode to start accepting live payments.
Integration tips:
- For more information, refer to our Quick Start Guide
- Follow our API Reference for more details about our API
- Use our Postman Collection to see the sample requests and responses
High-Level API Flow
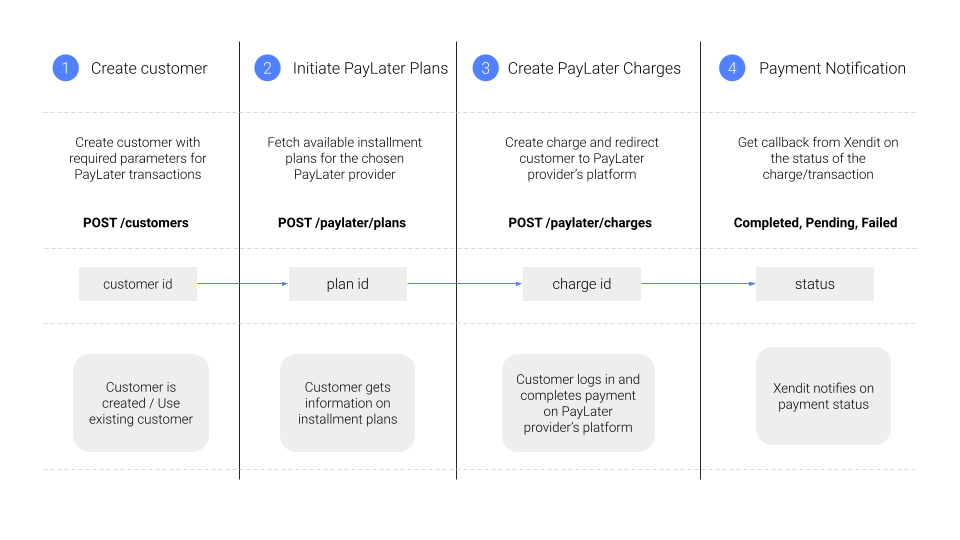
Create Customers
Customer objects are made in order to easily link transactions and payment methods to a certain customer.
INFO
PayLater Providers require you to include accurate details on customer, because it may be used to asses their credit risks, and issue refunds or chargebacks.
- Send a request of Create Customer API (API version 2020-10-31) with these required parameters:
given_names
surname
mobile_phone
email
addresses
country
street_line1
city
postal_code
- In the response, a customer id will be returned along with the details of the customer created
INFO
You only need to create a customer once so that future transactions can be connected to the specific customer (including for other PayLater charges, eWallets, Direct Debit, etc.
Sample create customer request
{
"reference_id" : "Gryffindor-student-003",
"given_names" : "Harry",
"surname" : "Potter",
"email" : "harry@hogwarts.co",
"mobile_number" : "+629991234567",
"addresses": [
{
"country": "ID",
"province": "Daerah Khusus Ibukota Jakarta",
"street_line1": "Jalan Makan",
"city": "Jakarta Pusat",
"postal_code": "12160"
}
]
}
Sample create customer response
{
"id": "e296f81b-074c-44ef-9977-d0ace6c6df1f",
"reference_id": "Gryffindor-student-003",
"given_names": "Harry",
"email": "harry@hogwarts.co",
"mobile_number": "+628234567890",
"description": null,
"middle_name": null,
"surname": "Potter",
"phone_number": null,
"nationality": null,
"date_of_birth": null,
"metadata": null,
"employment": null,
"addresses": [
{
"category": "",
"country": "ID",
"state": "",
"province": "Daerah Khusus Ibukota Jakarta",
"city": "Jakarta Pusat",
"postal_code": "12160",
"street_line1": "Jalan Makan",
"street_line2": "",
"is_preferred": false
}
]
}
Initiate PayLater Plans
Initiate Plans are used to provide your end-customer information on the available installment plans from the chosen PayLater provider, with the relevant amount and tenure.
Steps to create initiate PayLater plans:
- Send a POST request to Initiate PayLater Plans API with the required parameters. Use the
customer id
generated from Create Customer API. - In the response, available plans from the chosen PayLater provider will be displayed. The tenure will depend on the order amount and details you have provided in the request. A plan id will also be returned to be used in the next step (create charges).
Sample Simulate PayLater Plans
{
"customer_id": "e296f81b-074c-44ef-9977-d0ace6c6df1f",
"channel_code": "ID_KREDIVO",
"currency": "IDR",
"amount": 10000,
"order_items": [{
"type": "PHYSICAL_PRODUCT",
"reference_id": "SKU_backtoschool-promotion123",
"name": "Nymbus twothousand",
"net_unit_amount": 10000,
"quantity": 1,
"url": "https://www.wzardingworld.com/nymbus",
"category": "Sports",
"subcategory": "Equipment",
"description": "Sports equipment for quidditch"
}]
}
Sample Response
{
"id" : "plp_49915991-a7a8-4c21-9ae1-de9b95f5cb3d",
"customer_id": "e296f81b-074c-44ef-9977-d0ace6c6df1f",
"channel_code": "ID_KREDIVO",
"currency": "IDR",
"amount": 10000,
"order_items": [
{
"type": "PHYSICAL_PRODUCT",
"reference_id": "SKU_backtoschool-promotion123",
"name": "Nymbus twothousand",
"net_unit_amount": 10000,
"quantity": 1,
"url": "https://www.wzardingworld.com/nymbus",
"category": "Sports",
"subcategory": "Equipment",
"description": "Sports equipment for quidditch",
"metadata": null
}
],
"options": [
{
"total_amount": 11000,
"installment_amount": 11000,
"interval": "MONTH",
"interval_count": 1,
"total_recurrence": 1,
"interest_rate": 0,
"description": "Bayar dalam 30 hari"
}
],
"created": "2021-11-01T02:40:47.896Z"
}
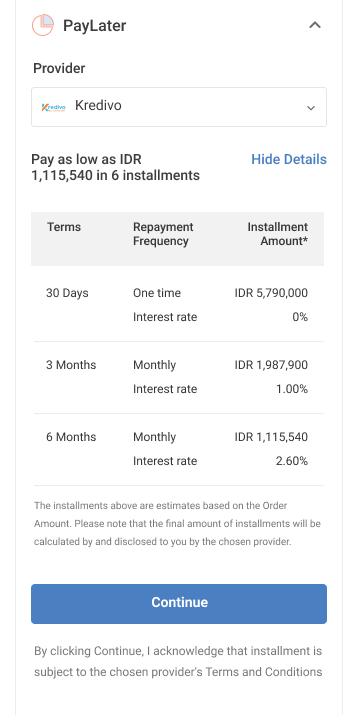
We recommend you put detailed installment plans as shown above to help your customers decide on the PayLater Providers and proceed to the payment completion. Your customer will choose their preferred PayLater plan and confirm the final amount for payment within the PayLater Provider’s platform.
INFO
The PayLater installment options are meant for reference only. These are estimates calculated by the Provider based on the order details in your API Request.
Order items
Take note of the mandatory parameters for order_items
. This should contain all the information from the customer's basket or shopping cart. Incomplete details will result in an error.
Type of Items:
PRODUCT
SERVICE
DIGITAL_PRODUCT
PHYSICAL_PRODUCT
DIGITAL_SERVICE
, andPHYSICAL_SERVICE
Required parameters:
reference_id
,name
,net_unit_amount
,quantity
,url
,category
Below is an illustration of how to compose the order_items
array based on the shopping cart.
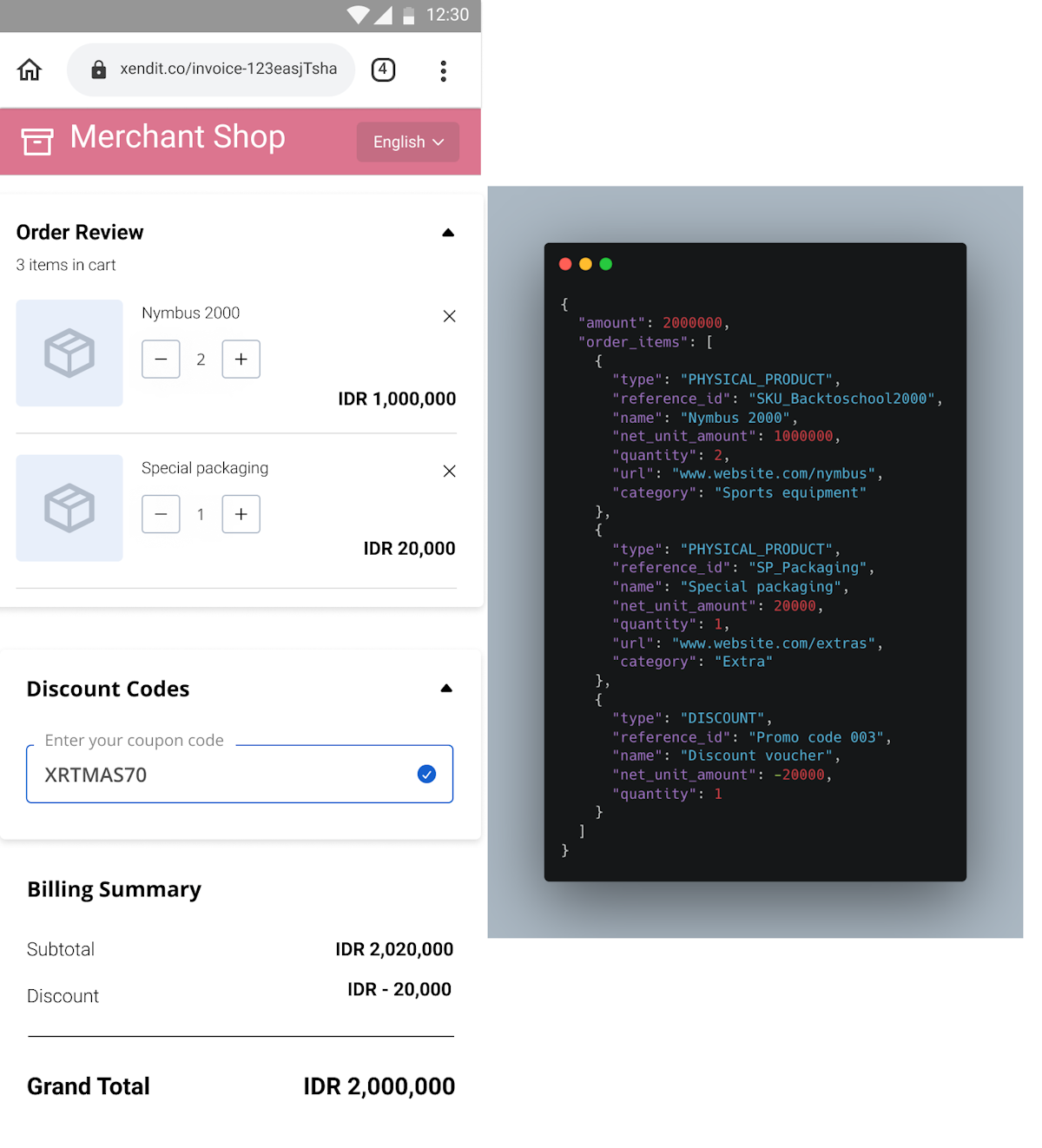
Create Charges (One-Time Payment)
INFO
Please set-up the callback URL in your Xendit dashboard (both in Test and Live mode) to accept payment status notifications and avoid errors
This request will charge the customer accordingly to complete payments in the chosen PayLater Provider’s platform. In this request, you can also set the URLs for customer when the charge succeeded or failed.
- Send a POST request to Create Charges API with the required parameters. Use the plan id generated from Initiate PayLater Plans API.
- In the response, the charge details with a
PENDING
status will be returned, along with the charge id and Checkout URL where customer can complete their payment. - Xendit will send a callback to the set callback URL once the PayLater charge status is confirmed
SUCCEEDED
Sample Create Charges Request
{
"plan_id": "plp_c0df725d-4997-461d-8530-f2cc661f7e14",
"reference_id": "order_id_456",
"checkout_method": "ONE_TIME_PAYMENT",
"success_redirect_url": "https://https://www.wzardingworld.com/successful-payments",
"failure_redirect_url": "https://https://www.wzardingworld.com/failed-payments"
}
Sample Create Charges Response
{
"id" : "plc_b7b2669e-0e3d-4cbd-8b36-cf16be78606c",
"business_id": "59ed61db656f544f3ca5v6f6",
"reference_id": "order_id_456",
"customer_id": "6a55adf8-1520-4651-8a05-9d04e0a4c48d",
"plan_id": "plp_c0df725d-4997-461d-8530-f2cc661f7e14",
"currency": "IDR",
"amount": 5000000,
"channel_code": "ID_KREDIVO",
"checkout_method": "ONE_TIME_PAYMENT",
"status": "PENDING",
"actions": {
"desktop_web_checkout_url": "https://pay-sandbox.kredivo.com/signIn?tk=c115175b-cf9c-445e-be24-3091a87e6316",
"mobile_web_checkout_url": "https://pay-sandbox.kredivo.com/signIn?tk=c115175b-cf9c-445e-be24-3091a87e6316"
},
"success_redirect_url": "https://www.wzardingworld.com/successful-payments",
"failure_redirect_url": "https://www.wzardingworld.com/failed-payments",
"callback_url": "https://webhook.site/5b30c7db-1873-4afd-b651-0ebaa16c4eee",
"created": "2021-10-29T06:50:57.877Z",
"updated": "2021-10-29T06:50:58.213Z",
"order_items": [
{
"type": "PHYSICAL_PRODUCT",
"reference_id": "SKU_123-456-789",
"name": "Nymbus 2000",
"net_unit_amount": 5000000,
"quantity": 1,
"url": "https://www.website.com/nymbus-2000",
"category": "Sports equipment",
"subcategory": "Quidditch",
"description": null,
"metadata": null
}
],
"voided_at": null,
"payment_method_id": null,
"metadata": null,
"channel_reference": "5cf2bf8a-b705-419a-9d2d-ad7cdf5363e2"
}
Refund PayLater Charge
Xendit will send you a callback / webhook for successful PayLater payments from your charge creation. Please ensure that you have set the Callback URL in the Xendit Dashboard for PayLater to receive it. Your customer will get their credit limit back in the PayLater Provider’s platform.
INFO
PayLater Refunds can only be done if the customer has paid the charge. Only available for KREDIVO, AKULAKU, and INDODANA.
INFO
Our refund APIs might also be useful to be integrated to your operational team’s dashboard.
- Send a POST request to Refund PayLater Charge API. Use the charge id of the payment to be refunded.
- In the response, the refund details with a status will be returned, along with the refund id.
Sample Refund PayLater Charge
{
"amount": 1000,
"reason": "DEFECTIVE_ITEM"
}
Sample Response PayLater Charge
{
"id" : "plr_2f2aa47f-2764-4b42-8712-c3fb1270b09e",
"charge_id" : "plc_8cb12305-9bcf-4441-a087-ee0d446e297b",
"channel_code" : "ID_KREDIVO",
"currency" : "IDR",
"amount" : 1000,
"reason" : "DEFECTIVE_ITEM",
"status" : "PENDING",
"created" : "2021-04-20T16:23:52Z",
"updated" : null
}
Last Updated on 2023-05-23