Create Recurring
You can create Recurring Payments via Xendit Dashboard or API depending on what might suit your business needs. To understand the benefits and the trade-off of choosing Xendit Dashboard or API, please go here
Create Recurring Payment via Dashboard
To create a Recurring Payment via dashboard, you Account User should have at least EDIT permission. Follow these steps to create a Recurring Payment via Xendit dashboard:
- Login to your Xendit dashboard
- Go to Accept Payments > Payment Link > Recurring
- Click "Create Recurring" to create a recurring and use the information below as your guide
Field | What to Fill |
---|---|
External ID | Unique identifier of an payment link in your system. There are no character restrictions for External ID. Create your identifier as you like Sample: HOUSE.AP/INV/XXV/341293TPR0004XJEDBDIEFDJIAGDY |
Amount per payment link | Amount that your customer will be charged at each interval of the recurring plan (can be in IDR, PHP or USD) |
Total Recurrence | The amount of time an payment link will be created within the same recurring plan in the time period you specify. Leave this field empty if you want the recurring plan to continue indefinitely |
Recurring Interval | The frequency with which a recurring payment payment link should be sent to your customer to pay within a recurring plan. It is the time period between when the last payment link and the next payment link are created, measured in days, weeks, or months |
payment link Due | Time period for which payment link is active and can be paid. payment links can be active for up to one year after creation |
Description | Short description for your end customer to read. You can use this free field to type notes or describe the purpose of the payment link Sample: Monthly membership fee for community gym |
Missed Payment Action | Indicates the behavior of the next recurring payment link if your customer missed the payment for the previous payment link. Setting 'Ignore' will continue to send your customer the next payment link at your specified interval even if they did not pay for the previous payment link before it expired. Setting 'Stop' will stop the recurring plan if your customer fails to pay for an payment link |
Autocharge | Clicking 'autocharge' will auto charge your customer’s debit/credit card in the next recurring interval. The recharge option is only available for card payments Autocharge option is provided for customers using cards. If a credit/debit card token is tied to a certain recurring payment plan, then every future payment for that particular recurring payment will automatically be charged right after the payment link is created |
Customer Information | Name, email address and phone number of your customer (the one you want to bill). You can set whether Xendit sends a payment link created, paid, and expired email to your customer on customize invoice |
Success Payment Redirect URL | The page you would like your customer to see if their payment is successful |
Failure Payment Redirect URL | The page you would like your customer to see if their payment has failed |
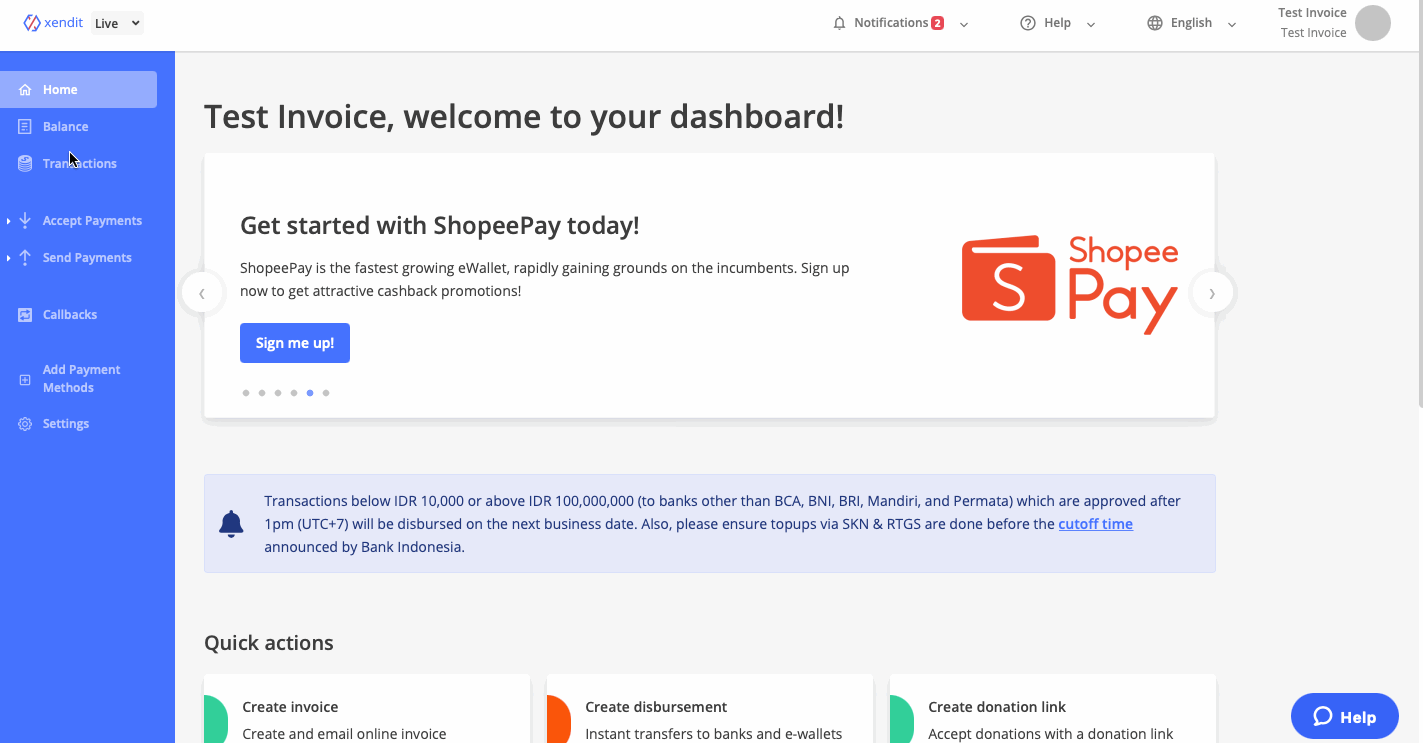
Create Recurring via API
Follow these steps to create an payment link via API:
- Follow the instruction in [](https://developers.xendit.co/api-reference/#create-payment link)Xendit API Reference on Create Recurring Payment section to start creating your recurring payment via API
- Setup your payment link Callback URL in Xendit Dashboard for "Invoice Paid"
Example of Create Recurring Payment via API
<?php
use Xendit\Xendit;
require 'vendor/autoload.php';
Xendit::setApiKey('xnd_development_P4qDfOss0OCpl8RtKrROHjaQYNCk9dN5lSfk+R1l9Wbe+rSiCwZ3jw==');
$params = [
'external_id' => 'recurring_31451441',
'payer_email' => 'sample_email@xendit.co',
'description' => 'Monthly room cleaning service',
'amount' => 125000,
'interval' => 'MONTH',
'interval_count' => 1
];
$createRecurring = \Xendit\Recurring::create($params);
var_dump($createRecurring);
?>
const x = new require('xendit-node')({ secretKey: 'xnd_development_P4qDfOss0OCpl8RtKrROHjaQYNCk9dN5lSfk+R1l9Wbe+rSiCwZ3jw==' });
const { RecurringPayment } = x;
const rpSpecificOptions = {};
const rp = new RecurringPayment(rpSpecificOptions);
const resp = await rp.createPayment({
externalID: 'recurring_31451441',
amount: 125000,
payerEmail: 'sample_email@xendit.co',
interval: RecurringPayment.Interval.Month,
intervalCount: 1,
description: 'Monthly room cleaning service',
});
console.log(resp);
Xendit.apiKey = "xnd_development_P4qDfOss0OCpl8RtKrROHjaQYNCk9dN5lSfk+R1l9Wbe+rSiCwZ3jw==";
try {
Map<String , Object> params = new HashMap<>();
params.put("external_id", "recurring_31451441");
params.put("payer_email", "sample_email@xendit.co");
params.put("interval", "MONTH");
params.put("interval_count", 1);
params.put("description", "Monthly room cleaning service");
params.put("amount", 125000);
RecurringPayment recurringPayment = RecurringPayment.create(params);
} catch (XenditException e) {
e.printStackTrace();
}
xendit.Opt.SecretKey = "xnd_development_P4qDfOss0OCpl8RtKrROHjaQYNCk9dN5lSfk+R1l9Wbe+rSiCwZ3jw=="
createData := recurringpayment.CreateParams{
ExternalID: "recurring_31451441",
Amount: 125000,
PayerEmail: "sample_email@xendit.co",
Description: "Monthly room cleaning service",
Interval: xendit.RecurringPaymentIntervalDay,
IntervalCount: 1,
}
resp, err := recurringpayment.Create(&createData)
if err != nil {
log.Fatal(err)
}
fmt.Printf("created recurring payment: %+v\n", resp)
from xendit import Xendit
api_key = "xnd_development_P4qDfOss0OCpl8RtKrROHjaQYNCk9dN5lSfk+R1l9Wbe+rSiCwZ3jw=="
xendit_instance = Xendit(api_key=api_key)
RecurringPayment = xendit_instance.RecurringPayment
recurring_payment = RecurringPayment.create(
external_id="recurring_12345",
payer_email="test@x.co",
description="Test Curring Payment",
amount=100000,
interval="MONTH",
interval_count=1,
)
print(recurring_payment)
Edit, Pause, Resume, and Stop Recurring Payment
Edit Recurring Payment
You can modify your Recurring Payment from Xendit Dashboard or API at any given time (as long as your Recurring Payment is not "Stopped") should you want to make alterations. To edit your Recurring Payment via Xendit Dashboard, go to Recurring Tab on the Xendit Dashboard, select your Recurring Payment, and click "Edit". You can modify almost all fields in the Create Recurring Payment except for the following:
- External ID
- Customer Information
- Credit Card Token
- Success Payment Redirect URL
- Failure Payment Redirect URL
To edit your Recurring Payment via API, please follow the Edit Recurring Payment API Reference.
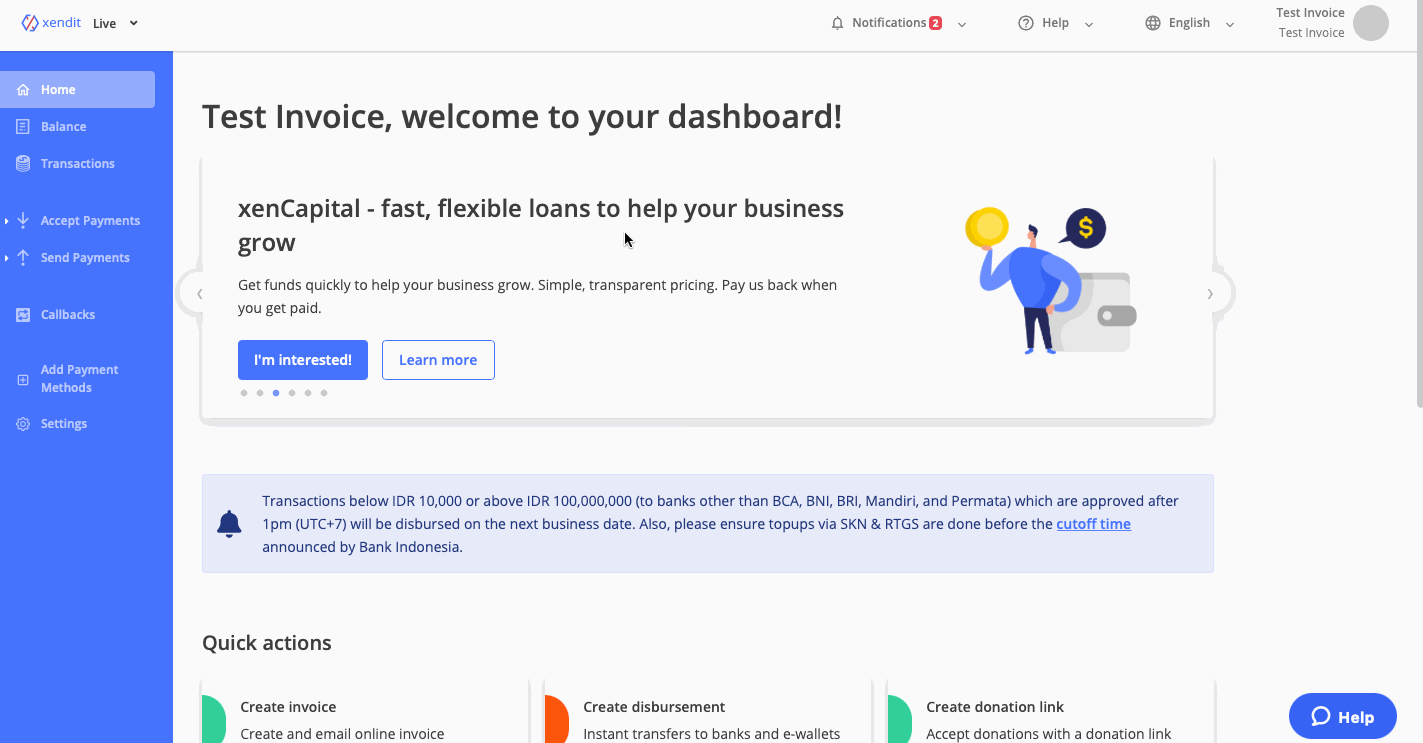
Pause and Resume Recurring Payment
You can pause and resume your Recurring Payment from Xendit Dashboard or API at any given time (as long as your Recurring Payment is not "Stopped") should you want to stop the recurring schedule temporarily and reactivate again in the future. To pause and resume your Recurring Payment via Xendit Dashboard, go to Recurring Tab on the Xendit Dashboard, select your Recurring Payment, and click "Pause" or "Resume".
To pause your Recurring Payment via API, please follow the Pause Recurring Payment API Reference, and to resume it, please follow the Resume Recurring Payment API Reference.
Note: If your Recurring Payment's status is Active, "Resume" button will not show up as an option in the Xendit Dashboard. On the other hand, if your Recurring Payment's status is already Paused, "Pause" button will not show up.
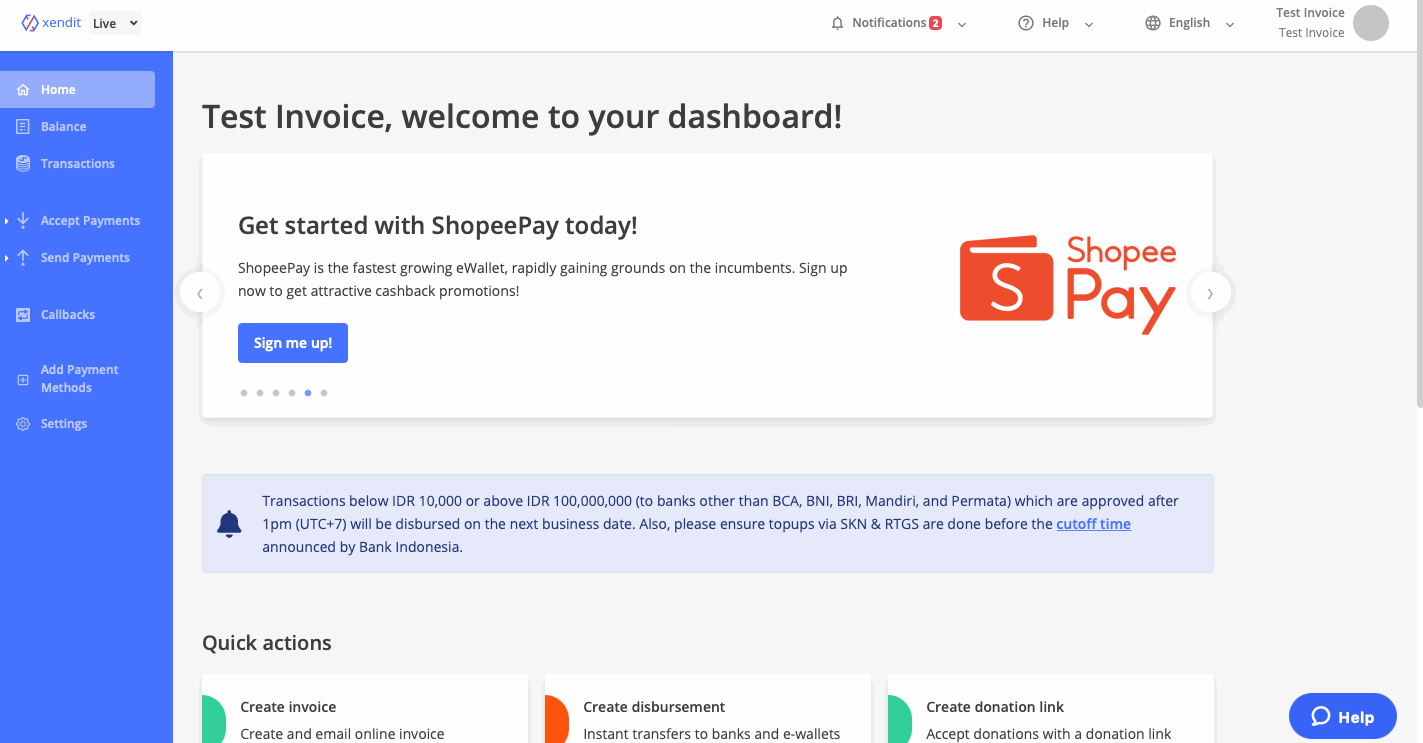
Stop Recurring Payment
If you wish to stop your Recurring Payment altogether, you can also do it via Xendit Dashboard or API. To stop your Recurring Payment via Xendit Dashboard, go to Recurring Tab on the Xendit Dashboard, select your Recurring Payment, and click "Stop".
To stop your Recurring Payment via API, please follow the Stop Recurring Payment API Reference.
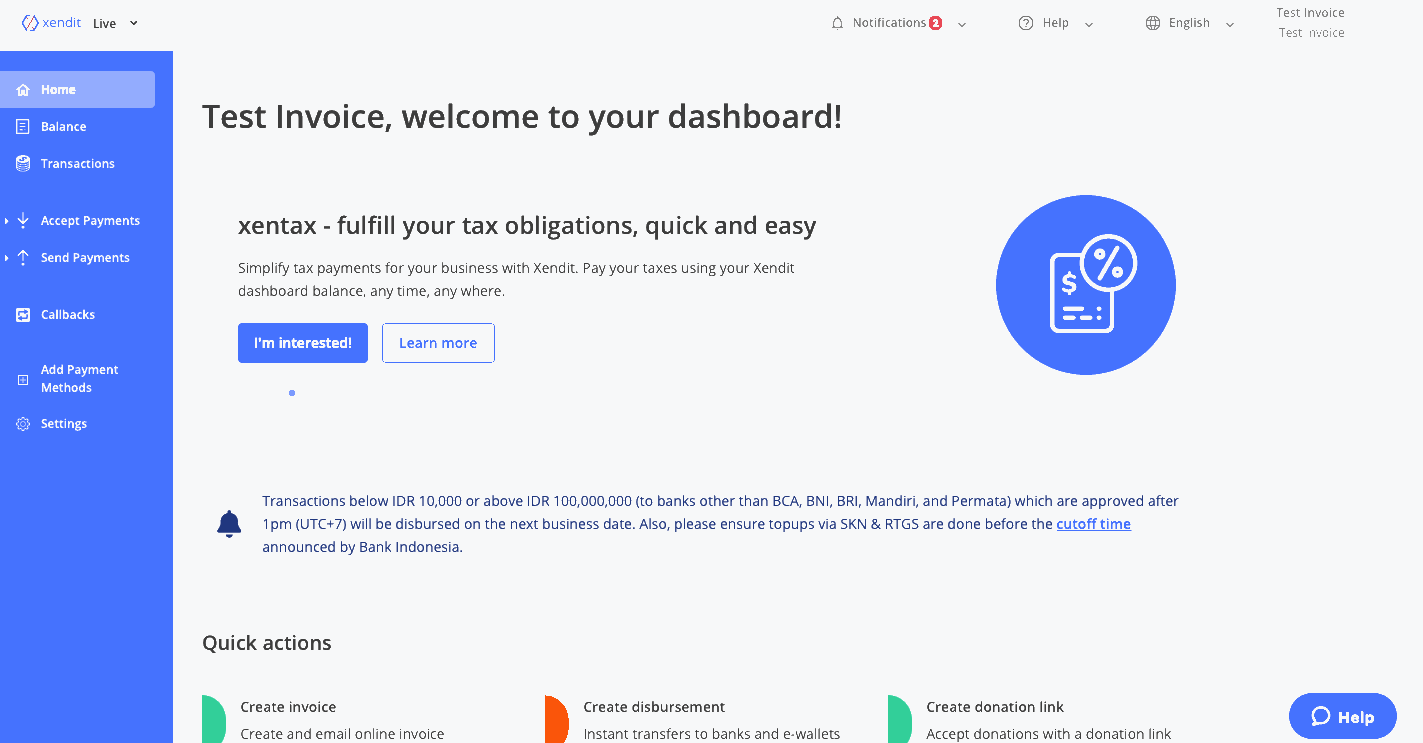
Last Updated on 2023-05-24